Software Design Patterns
Florian Rappl, Fakultät für Physik, Universität Regensburg
Software Design Patterns
Introduction to modern software architecture
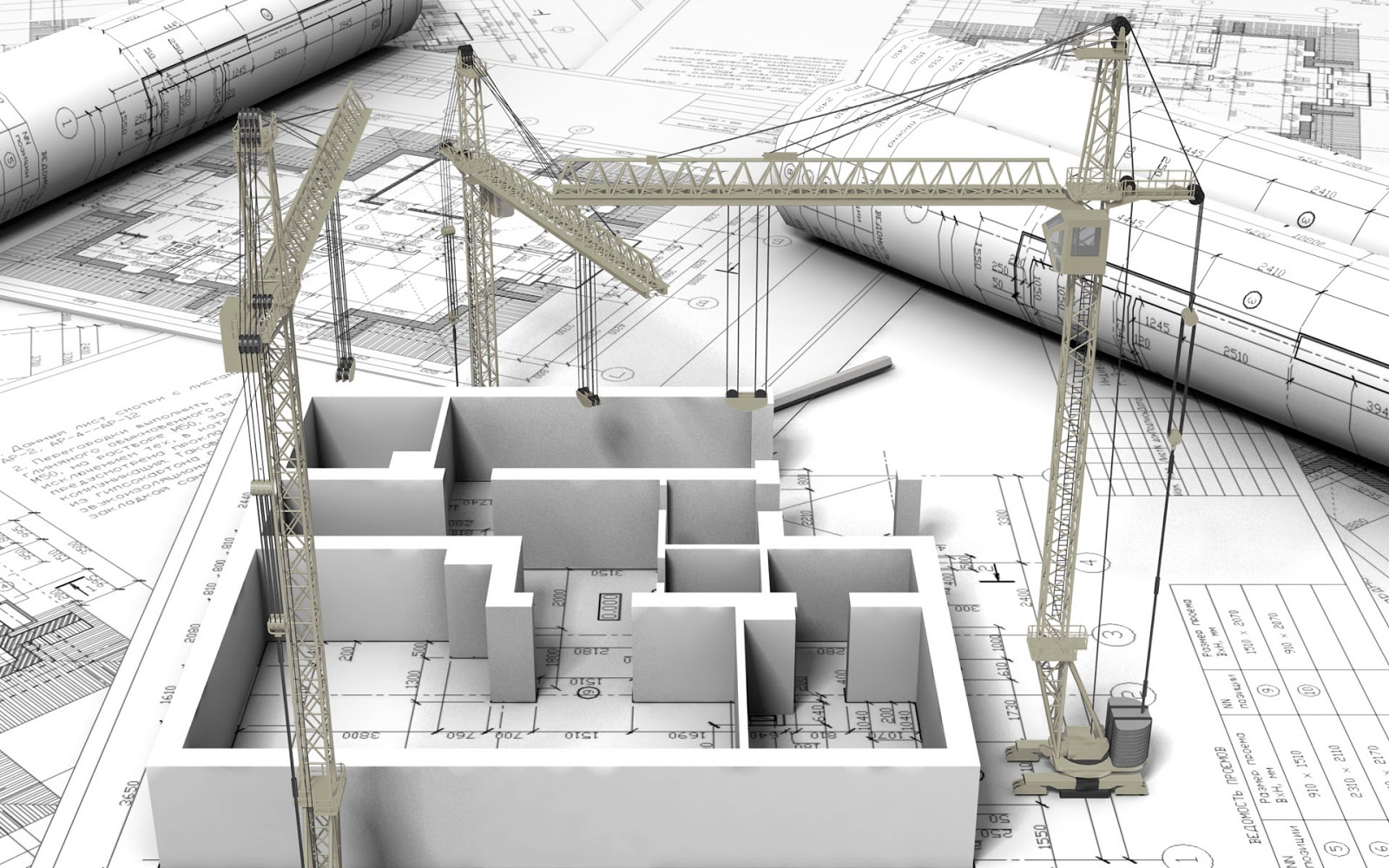
Software Life Cycle
Software development processes
- A software development life-cycle (short SDLC) structures development
- This is often called software development process
- We can categorize various different approaches by their attitude towards:
- Planning
- Implementation, testing and documenting
- Deployment and maintenance
- Most of this is only of academic interest
Planning
- A crucial phase as requirements are set
- Also a draft for the software specification is created
- Better planning enables a better (faster, bug-free and feature-rich) development, but requires a lot experience
- Sometimes planning can also result in contracts and legal documents (especially for individual software)
- The requirement analysis can also contain prototype creation
Implementation
- Actually writing code with logic is called implementation
- This phase could be broken in various sub-phases (prototype, API, realization, ...)
- Usually the whole process should divided into little tasks
- Developers take care of these little tasks
- Engineers keep in mind the big picture
Testing
- Testing is important to ensure quality
- Goal: Minimizing bugs, maximizing usability and stability
- There are multiple kind of tests: automatic, manual, usability, performance, ...
- Some of them are contradictions (there cannot be automatic usability tests), some of them should be combined (like automatic code unit tests)
- There are movements towards dictating the implementation by automatic tests (see TDD chapter)
Documentation
- Documentation is important due to various reasons
- Manuals for users (top level)
- Manuals for other developers (low level)
- Legal documents (fulfillment)
- Finding bugs and improving code
- Documentation should not only be done in documents but also in comments in the code
- Many tools can take comments and create documents
Deployment
- Finally a software product can be released
- More than just releasing a binary might be required:
- Installation
- Documentation
- Customization
- Testing and evaluating
- Training and support might demanded as well
Maintenance
- Software is never finished
- Updates may be needed due to include new features or fix bugs
- Here we are usually out of the requirement analysis scope
- This is where excellent software architecture shines
- Making software maintainable is the hardest task
Existing models
- Waterfall model
- Spiral model
- Iterative and incremental development
- Agile development
- Rapid application development
Waterfall model
Predictive and adaptive planning
- Desire for predictability in software development
- Predictive planning uses two stages:
- Coming up with a plans (difficult to predict)
- Executing the plans (predictable)
- However, majority suffers from a requirement churn
- Let's face it: the requirement churn cannot be avoided
- Now we are free to do adaptive planning, i.e. trying to deliver the best software and react to changes
Iterative model
Waterfall Vs Iterative
- Both break a project into smaller chunks
- Waterfall does it based on activity
- Iterative identifies subsets of functionality
- No one has ever followed one of those purely
- There will always be impurities leaking into the process
- Iterative development can also appear as incremental, spiral, evolutionary etc.
Staged delivery
- Pure waterfall has never been used and waterfall is generally disliked
- One can have an hybrid approach that takes a subset of waterfall and the iterative process
- Analysis and high-level design are usually done first
- Coding and testing phases are then executed in an iterative manner
- Main advantage with iterative coding: Better indication of problems
- Consider using time boxing to force iterations to be a fixed length of time
Agile software development
Agile processes
- Agile is an umbrella term that covers many processes
- Examples: Extreme Programming, Scrum, Feature Driven Development
- Very adaptive in their nature
- Quality of people and their interaction results in quality of code
- Agile methods tend to use short, time-boxed iterations (a month or less)
- Usually agile is considered lightweight
Extreme Programming
Rational Unified Process
- A process framework
- Provides vocabulary and structure for discussing processes
- A development case needs to be selected
- RUP is essentially an iterative process and has four phases:
- Inception (initial evaluation)
- Elaboration (identifying requirements)
- Construction (implementation)
- Transition (late-stage activities)
Techniques
- Developing software efficiently is not only a matter of the planned life cycle, but also of using techniques like
- Version controlling (e.g. git)
- Automated regression tests (e.g. JUnit)
- Refactoring (e.g. VS)
- Continuous integration (e.g. TFS)
- We will have a look at two of those techniques later
Literature
- Fowler, Martin (2003). UML Distilled: A Brief Guide to the Standard Object Modeling Language (3rd ed.).
- McConnell, Steve (1996). Rapid development: taming wild software schedules.
- Kent, Beck (2000). Extreme Programming.
- Highsmith, James (1999). Adaptive Software Development.